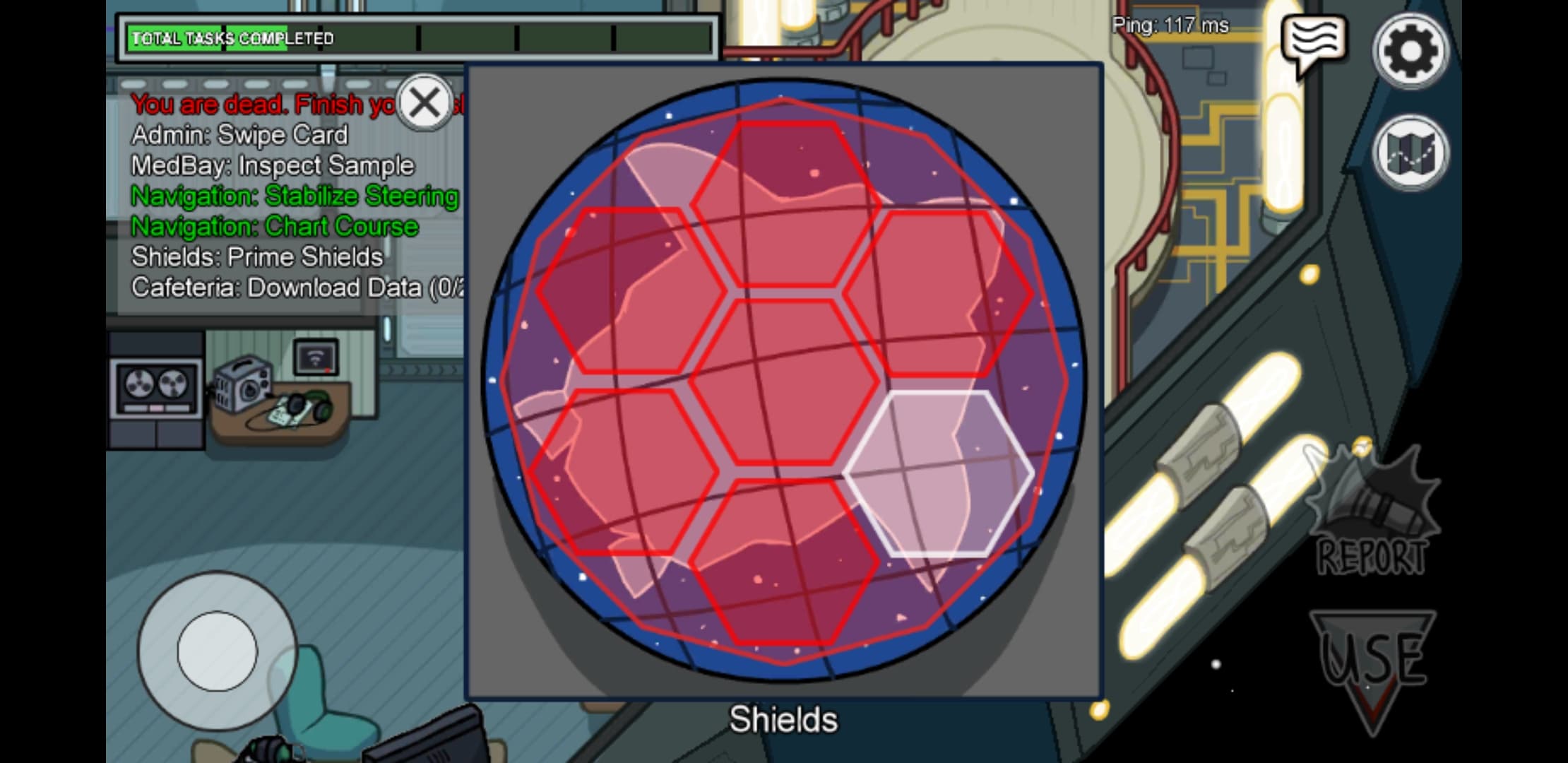
Let’s show you how to make the shields task in unity from Among Us. This series is focusing on the mini-game/ mini-task portion of Among Us. We are building the level and character movement in Among Us with the series located here: https://www.infogamerhub.com/among-us-lesson-1/
The task that we are focusing on in this post is the Shields task/mini-game. The image above is for reference.
If you are wondering why the end result doesn’t look as pretty as the reference image it is because I didn’t spend the time to make it look exactly like it (reasoning for this is due to laziness and the rest is due to be a quicker prototype)(You might notice the missing turtle feet, whoops :)). I wanted to make sure to cover the overall functionality of the task was correct. We do recommend You change the task up to look and behave a little differently if you are to implement this in your own game.
How To Make Among Us Task in Unity – Shields
Attention Members!
The second half of this post will show how to make the game more difficult and it is for members only. We hope if you are not a member you will try us out for the extra support and content. The second part of this post for members is coming soon!
Become A Member
We are making tons of helpful content for game developers! Have we helped you before? If we have, help us in return by becoming a member. We are keeping the membership cost low to create maximum value and affordability for members.
Hierarchy and Inspectors:


How To Make Among Us – Shields Script:
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class Shields : MonoBehaviour { [SerializeField] GameObject[] shieldButtons; // array to hold all buttons [SerializeField] int[] shieldButtonsInt; // int array for holding the random numbers. [SerializeField] bool Failed = true; [SerializeField] GameObject shieldGamePanel; [SerializeField] GameObject taskCompleted; [SerializeField] GameObject dodecagon; [SerializeField] Image imageHolder; Color32 red = new Color32(255, 39, 0, 255); Color32 green = new Color32(4, 204, 0, 255); Color32 invisible = new Color32(4, 204, 0, 0); Color32 white = new Color32(255, 255, 255, 255); Color32 clearWhite = new Color32(255, 255, 255, 105); // last number in the series (105) is for alpha channel or the opacity of the image where 255 is solid and 0 is invisible. Color32 clearRed = new Color32(255, 39, 0, 105); private void OnEnable() { EnableInteractableButtons(); taskCompleted.SetActive(false); dodecagon.GetComponent<Image>().color = red; dodecagon.transform.GetChild(0).GetComponent<Image>().color = clearRed; for (int i = 0; i < shieldButtons.Length; i++) { shieldButtonsInt[i] = (Random.Range(0, 2)); //Debug.Log("This Gets Run"); } for (int i = 0; i < shieldButtons.Length; i++) { if (shieldButtonsInt[i] == 0) { shieldButtons[i].GetComponent<Image>().color = white; //If your image is only one image you won't have to do the second part //shieldButtons[i].transform.GetComponentInChildren<Image>().color = white; //For some reason this wasn't getting the child component. shieldButtons[i].transform.GetChild(0).GetComponent<Image>().color = clearWhite; //So I did this instead // Debug.Log("for loop" + lightOrder[i]); } if (shieldButtonsInt[i] == 1) { shieldButtons[i].GetComponent<Image>().color = red; shieldButtons[i].transform.GetChild(0).GetComponent<Image>().color = clearRed; //shieldButtons[i].transform.GetComponentInChildren<Image>().color = red; } } } public void ShieldButtonsClicked(int button) { if (shieldButtons[button].GetComponent<Image>().color == red) { shieldButtons[button].GetComponent<Image>().color = white; shieldButtons[button].transform.GetChild(0).GetComponent<Image>().color = clearWhite; } else { shieldButtons[button].GetComponent<Image>().color = red; //shieldButtons[button].GetComponentInChildren<Image>().color = red; shieldButtons[button].transform.GetChild(0).GetComponent<Image>().color = clearRed; } AreAllButtonsWhite(); } public void AreAllButtonsWhite() { Failed = false; for (int i = 0; i < shieldButtons.Length; i++) { if (shieldButtons[i].GetComponent<Image>().color == red) { Failed = true; break; } } if (Failed == false) { DisableInteractableButtons(); Debug.Log("You Won"); dodecagon.GetComponent<Image>().color = white; dodecagon.transform.GetChild(0).GetComponent<Image>().color = clearWhite; taskCompleted.SetActive(true); Invoke("ClosePanel",1); } } public void ClosePanel() { shieldGamePanel.SetActive(false); } public void OpenPanel() { shieldGamePanel.SetActive(true); } void DisableInteractableButtons() { for (int i = 0; i < shieldButtons.Length; i++) { shieldButtons[i].GetComponent<Button>().interactable = false; } } void EnableInteractableButtons() { for (int i = 0; i < shieldButtons.Length; i++) { shieldButtons[i].GetComponent<Button>().interactable = true; } } }
Make Among Us Extra Content For Members
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }
Let Us Know How We Did
Were you able to learn how to make the shields task in unity from Among Us? We are wanting to always make our content more helpful and we can’t do it without your input. Please leave us a comment on this post’s YouTube video located here: https://youtu.be/GJGOKur3kB0 let us know how we did. If there are other ideas on how to use the code or better ways to implement the code in this video also let us know at the same location.