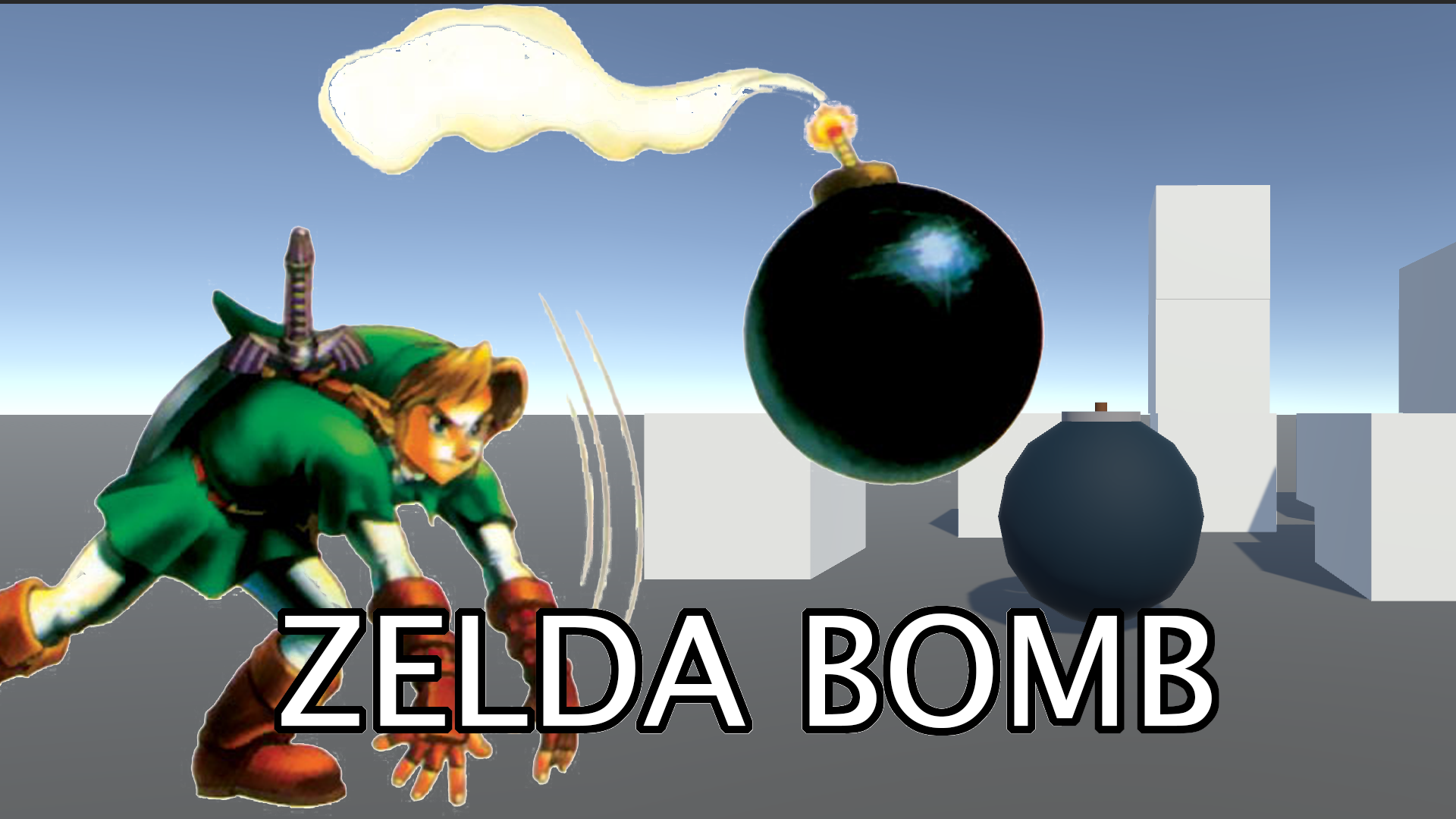
Welcome to our Unity video tutorials on how to create the Zelda bomb mechanic and prefab as seen in the classic game, Zelda Ocarina of Time.
In this tutorial, we’ll guide you through the process of creating the bomb mechanic, which involves creating a bomb that could be picked up and thrown by the player. We’ll also show you how to create a blink effect and bomb explosion. This prefab can be easily integrated into your game projects.
Our tutorials will cover a range of topics, including how to create the bomb object using Blender’s 3D modeling tools, how to program the bomb physics and behaviors using C#, and how to create the bomb explosion effect using particle effects and sound effects.
We’ll also demonstrate how to create a bomb prefab that can be easily dropped into any game project, making it simple to add the Zelda bomb mechanic to your own games.
Throughout the tutorial, we’ll provide clear explanations and demonstrations, making it easy for even beginners to follow along. We’ll also offer tips and tricks to help you optimize your bomb mechanic and make it as engaging and fun as possible.
By the end of this tutorial, you’ll have a complete Zelda bomb mechanic and prefab that you can integrate into your own game projects or use as a starting point for creating your own unique game mechanics.