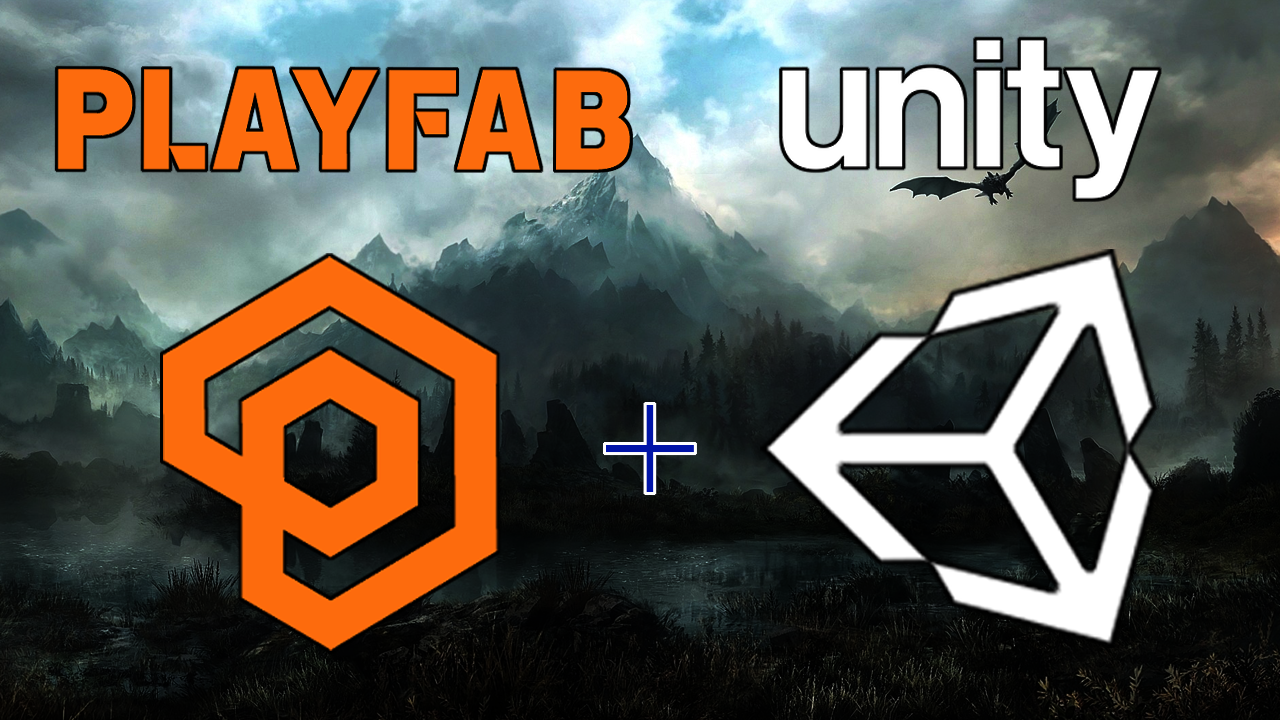
Create a Mobile Login System
For this lesson, we will be teaching you how to log in and register players in your game using a mobile device ID. This is all done with Unity 3D and the PlayFab SDK. There are two types of logins with the PlayFab SDK, anonymous and recovery logins. Anonymous logins do not require any user input to register the play, only the device ID. Recovery logins require some form of identification provided by the user, such as usernames and passwords.
Tutorial Setup
Welcome back to Info Game, This is the third lesson in our PlayFab tutorial series. We will teach you how to better you the PlayFab plugin with Unity 3D. In this last lesson, we taught you how to register and login a player using their username and password. In this video, we will expound on this and teach you how to Register players and log them in using mobile devices.
Before we get started you should take a look at your PlayFab backend dashboard. See if you were able to register new players from what we did in the last lesson. First, you will need to login to your PlayFab dashboard. You can find this by selecting your game in the PlayFab dashboard then select Players from the left-hand column. On the next page, you should be able to see a list of all your players.
Anonymous Logins
Now we will start coding our lesson. We will first want to open up our PlayFabLogin script. Once opened we will begin by creating an anonymous login for Android and iOS devices. This is good to have in your game because they do not require the players to give any personal information to create an account. The only information we need is the device ID which can be accessed in your login script. We will be creating this anonymous login in our start function. We will also use platform-dependent regions to create code for both Android and iOS.
Recoverable Logins
Once we have finished creating these anonymous logins we will then create a way for players to add there person login information so that their anonymous login becomes a recoverable login account.
Working in Unity
In Unity, we will duplicate our login panel and use this new panel to have players add the login information to their anonymous account. We will then create a new button that will bring up this new panel when a player clicks it.
Testing your Project
After you have finished adding all this functionality it is time to test our game. To test the mobile logins you can build your project on to an Android device. Your device should be automatically registered when the application first opens. You can check this by going back to your PlayFab dashboard and look to see if you have a new Android entry. You can then try adding a username and password to your Android account. Then go to your dashboard one more time and select your Android account and see if the same username has been added to the account.
If you are able to get all of this to work then you have done it correctly. This is everything we will talk about in this lesson. Make sure you like this video and leave any questions you have in the comments below. Also, subscribe to our channel so you can be up to date with all our content.
Watch how to Create a Mobile Login
PlayFabLogin.cs
using PlayFab; using PlayFab.ClientModels; using UnityEngine; using PlayFab.DataModels; using PlayFab.ProfilesModels; using System.Collections.Generic; public class PlayFabLogin: MonoBehaviour { private string userEmail; private string userPassword; private string username; public GameObject loginPanel; public GameObject addLoginPanel; public GameObject recoverButton; public void Start() { //Note: Setting title Id here can be skipped if you have set the value in Editor Extensions already. if (string.IsNullOrEmpty(PlayFabSettings.TitleId)) { PlayFabSettings.TitleId = "8741"; // Please change this value to your own titleId from PlayFab Game Manager } //PlayerPrefs.DeleteAll(); //var request = new LoginWithCustomIDRequest { CustomId = "GettingStartedGuide", CreateAccount = true }; //PlayFabClientAPI.LoginWithCustomID(request, OnLoginSuccess, OnLoginFailure); if (PlayerPrefs.HasKey("EMAIL")) { userEmail = PlayerPrefs.GetString("EMAIL"); userPassword = PlayerPrefs.GetString("PASSWORD"); var request = new LoginWithEmailAddressRequest { Email = userEmail, Password = userPassword }; PlayFabClientAPI.LoginWithEmailAddress(request, OnLoginSuccess, OnLoginFailure); } else { #if UNITY_ANDROID var requestAndroid = new LoginWithAndroidDeviceIDRequest { AndroidDeviceId = ReturnMobileID(), CreateAccount = true }; PlayFabClientAPI.LoginWithAndroidDeviceID(requestAndroid, OnLoginMobileSuccess, OnLoginMobileFailure); #endif #if UNITY_IOS var requestIOS = new LoginWithIOSDeviceIDRequest { DeviceId = ReturnMobileID(), CreateAccount = true }; PlayFabClientAPI.LoginWithIOSDeviceID(requestIOS, OnLoginMobileSuccess, OnLoginMobileFailure); #endif } } #region Login private void OnLoginSuccess(LoginResult result) { Debug.Log("Congratulations, you made your first successful API call!"); PlayerPrefs.SetString("EMAIL", userEmail); PlayerPrefs.SetString("PASSWORD", userPassword); loginPanel.SetActive(false); recoverButton.SetActive(false); } private void OnLoginMobileSuccess(LoginResult result) { Debug.Log("Congratulations, you made your first successful API call!"); loginPanel.SetActive(false); } private void OnRegisterSuccess(RegisterPlayFabUserResult result) { Debug.Log("Congratulations, you made your first successful API call!"); PlayerPrefs.SetString("EMAIL", userEmail); PlayerPrefs.SetString("PASSWORD", userPassword); loginPanel.SetActive(false); } private void OnLoginFailure(PlayFabError error) { var registerRequest = new RegisterPlayFabUserRequest { Email = userEmail, Password = userPassword, Username = username }; PlayFabClientAPI.RegisterPlayFabUser(registerRequest, OnRegisterSuccess, OnRegisterFailure); } private void OnLoginMobileFailure(PlayFabError error) { Debug.Log(error.GenerateErrorReport()); } private void OnRegisterFailure(PlayFabError error) { Debug.LogError(error.GenerateErrorReport()); } public void GetUserEmail(string emailIn) { userEmail = emailIn; } public void GetUserPassword(string passwordIn) { userPassword = passwordIn; } public void GetUsername(string usernameIn) { username = usernameIn; } public void OnClickLogin() { var request = new LoginWithEmailAddressRequest { Email = userEmail, Password = userPassword }; PlayFabClientAPI.LoginWithEmailAddress(request, OnLoginSuccess, OnLoginFailure); } public static string ReturnMobileID() { string deviceID = SystemInfo.deviceUniqueIdentifier; return deviceID; } public void OpenAddLogin() { addLoginPanel.SetActive(true); } public void OnClickAddLogin() { var addLoginRequest = new AddUsernamePasswordRequest { Email = userEmail, Password = userPassword, Username = username }; PlayFabClientAPI.AddUsernamePassword(addLoginRequest, OnAddLoginSuccess, OnRegisterFailure); } private void OnAddLoginSuccess(AddUsernamePasswordResult result) { Debug.Log("Congratulations, you made your first successful API call!"); PlayerPrefs.SetString("EMAIL", userEmail); PlayerPrefs.SetString("PASSWORD", userPassword); addLoginPanel.SetActive(false); } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }