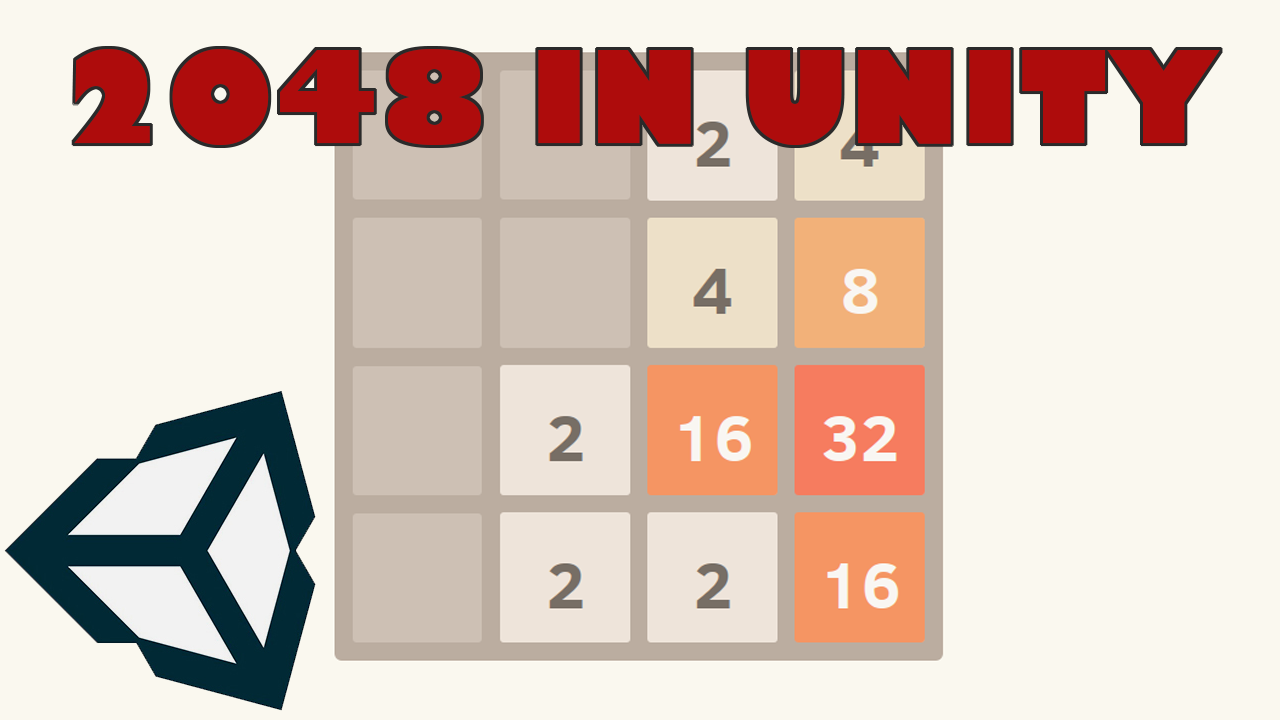
For this lesson on how to make 2048 in Unity, we will create an action event that will send a message to each of our cell scripts when the player inputs a direction or WASD. This is in preparation for getting the fill blocks to move and combine when they are shifted.
GameController2048.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using System; public class GameController2048 : MonoBehaviour { [SerializeField] GameObject fillPrefab; [SerializeField] Transform[] allCells; public static Action<string> slide; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if(Input.GetKeyDown(KeyCode.Space)) { SpawnFill(); } if(Input.GetKeyDown(KeyCode.W)) { slide("w"); } if (Input.GetKeyDown(KeyCode.D)) { slide("d"); } if (Input.GetKeyDown(KeyCode.S)) { slide("s"); } if (Input.GetKeyDown(KeyCode.A)) { slide("a"); } } public void SpawnFill() { int whichSpawn = UnityEngine.Random.Range(0, allCells.Length); if(allCells[whichSpawn].childCount != 0) { Debug.Log(allCells[whichSpawn].name + " is already filled"); SpawnFill(); return; } float chance = UnityEngine.Random.Range(0f, 1f); Debug.Log(chance); if(chance <.2f) { return; } else if(chance < .8f) { GameObject tempFill = Instantiate(fillPrefab, allCells[whichSpawn]); Fill2048 tempFillComp = tempFill.GetComponent<Fill2048>(); tempFillComp.FillValueUpdate(2); } else { GameObject tempFill = Instantiate(fillPrefab, allCells[whichSpawn]); Fill2048 tempFillComp = tempFill.GetComponent<Fill2048>(); tempFillComp.FillValueUpdate(4); } } }
Cell2048.cs
using System; using System.Collections; using System.Collections.Generic; using UnityEngine; public class Cell2048 : MonoBehaviour { public Cell2048 right; public Cell2048 down; public Cell2048 left; public Cell2048 up; private void OnEnable() { GameController2048.slide += OnSlide; } private void OnDisable() { GameController2048.slide -= OnSlide; } private void OnSlide(string whatWasSent) { Debug.Log(whatWasSent); } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }