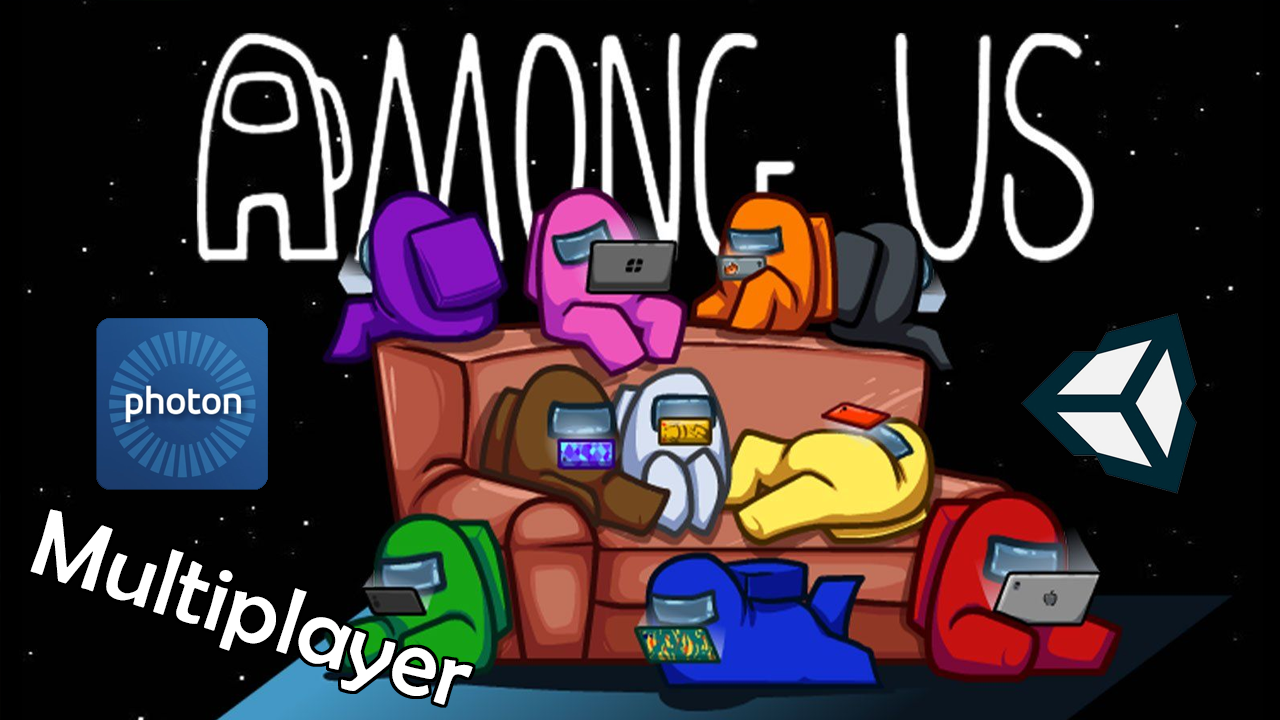
For this lesson on how to create a multiplayer Among Us game in Unity, we will show you how to pick and sync an imposter across the network. Having a random imposter is vital for our among us game as the imposter is what makes the game challenging and gives the game an opposition.
First, we will pick a random number between zero and the number of players in our room. We will then send that number to all the clients and whichever client is that number in the room will become the imposter. That player will then be able to kill the other players.
AU_SpawnPoints.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public class AU_SpawnPoints : MonoBehaviour { public static AU_SpawnPoints instance; public Transform[] spawnPoints; // Start is called before the first frame update void Start() { instance = this; } }
MyPhotonPlayer.cs
using Photon.Pun; using Photon.Realtime; using System.Collections; using System.Collections.Generic; using System.IO; using UnityEngine; public class MyPhotonPlayer : MonoBehaviour { PhotonView myPV; GameObject myPlayerAvatar; Player[] allPlayers; int myNumberInRoom; // Start is called before the first frame update void Start() { myPV = GetComponent<PhotonView>(); allPlayers = PhotonNetwork.PlayerList; foreach(Player p in allPlayers) { if(p!= PhotonNetwork.LocalPlayer) { myNumberInRoom++; } } if (myPV.IsMine) { myPlayerAvatar = PhotonNetwork.Instantiate(Path.Combine("PhotonPrefabs", "AU_Player"), AU_SpawnPoints.instance.spawnPoints[myNumberInRoom].position, Quaternion.identity); } } }
AU_GameController.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; public class AU_GameController : MonoBehaviour { PhotonView myPV; int whichPlayerIsImposter; // Start is called before the first frame update void Start() { myPV = GetComponent<PhotonView>(); if(PhotonNetwork.IsMasterClient) { PickImposter(); } } void PickImposter() { whichPlayerIsImposter = Random.Range(0, PhotonNetwork.CurrentRoom.PlayerCount); myPV.RPC("RPC_SyncImposter", RpcTarget.All, whichPlayerIsImposter); Debug.Log("Imposter " + whichPlayerIsImposter); } [PunRPC] void RPC_SyncImposter(int playerNumber) { whichPlayerIsImposter = playerNumber; AU_PlayerController.localPlayer.BecomeImposter(whichPlayerIsImposter); } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }