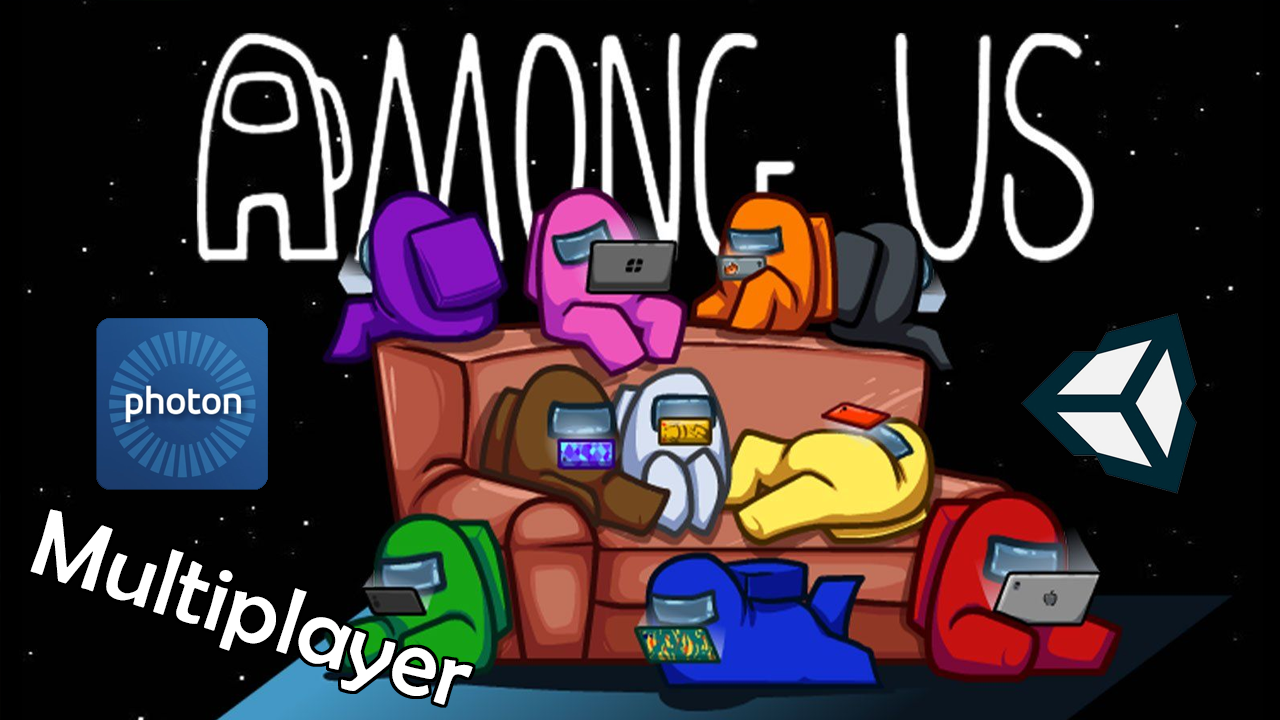
For this lesson on how to create Among Us in Unity using Photon, I will show you how to create Scene Navigation so you can transition from the main menu scene to the waiting room scene and then to the game scene. This is an important feature that we need to cover before we can show you other game mechanics like how to pick the imposter.
To do this we will have the quick start menu load the player first into the waiting room scene. Then when all the players have loaded in the host of the game will have a button that will begin a timer. When the timer reaches zero it will then load all the players into the game scene.
WaitingRoomController.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; using UnityEngine.UI; public class WaitingRoomController : MonoBehaviour { PhotonView myPV; [SerializeField] float timeToStart; float timerToStart; bool readyToStart; [SerializeField] GameObject StartButton; [SerializeField] Text countDownDisplay; [SerializeField] int nextLevel; // Start is called before the first frame update void Start() { myPV = GetComponent<PhotonView>(); timerToStart = timeToStart; } // Update is called once per frame void Update() { StartButton.SetActive(PhotonNetwork.IsMasterClient); if(readyToStart) { timerToStart -= Time.deltaTime; countDownDisplay.text = ((int)timerToStart).ToString(); } else { timerToStart = timeToStart; countDownDisplay.text = ""; } if (PhotonNetwork.IsMasterClient) { if (timerToStart <= 0) { timerToStart = 100; PhotonNetwork.AutomaticallySyncScene = true; PhotonNetwork.LoadLevel(nextLevel); } } } public void Play() { if(PhotonNetwork.IsMasterClient) { myPV.RPC("RPC_Play", RpcTarget.All); } } [PunRPC] void RPC_Play() { readyToStart = !readyToStart; } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }