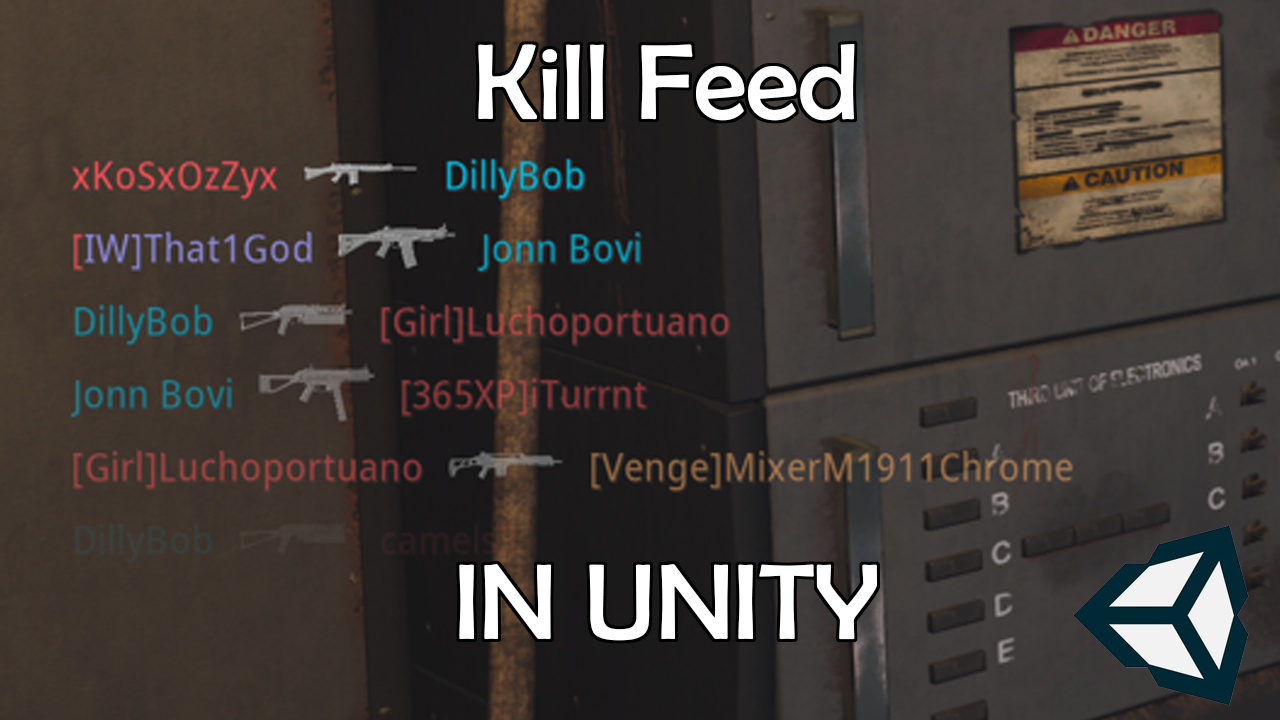
For this lesson on how to create game mechanics for Unity, I will show you how to create a Kill Feed UI as found in most first-person shooters and other fighting games. By the end of this tutorial, you will have a working kill feed prefab that you can add to any Unity project. This prefab is also very easy to use. Just add it to any scene you which to use it in and then there are three options for using this mechanic. You can have if display just names, names and how, or names and an icon of how each player was kill. There is then a public function for each of these options that you can call from anywhere in your project using the singleton of the prefab.
Kill Feed Prefab
This is a simple prebuild prefab that will add an easy to use kill feed to any Unity project you want.
KillListing.cs
using UnityEngine; using UnityEngine.UI; //This script is attached to the kill listing prefab public class KillListing : MonoBehaviour { [SerializeField] Text killerDisplay; //The UI Text that displays the killer name [SerializeField] Text killedDisplay; //The UI Text that displays the Killed name [SerializeField] Text howDisplay; [SerializeField] Image howImageDisplay; private void Start() { Destroy(gameObject, 10f); //To keep our feed from getting too long we destroy old listings after a given time. } //This function is called by the AddNewKillListing function to set the two text variables. public void SetNames(string killerName, string killedName) { killerDisplay.text = killerName; killedDisplay.text = killedName; } public void SetNamesAndHow(string killerName, string killedName, string how) { killerDisplay.text = killerName; killedDisplay.text = killedName; howDisplay.text = how; } public void SetNamesAndHowImage(string killerName, string KilledName, Sprite howImage) { killerDisplay.text = killerName; killedDisplay.text = KilledName; howImageDisplay.sprite = howImage; } }
KillFeed.cs
using UnityEngine; //This script is attached to the Kill Feed object and is used to control the feed. public class KillFeed : MonoBehaviour { public static KillFeed instance; //Singleton of this script so you can call the AddNewKillListing function from anywhere in your project. [SerializeField] GameObject killListingPrefab; //This is a variable to hold the kill listing prefab. [SerializeField] GameObject killListingWithImagePrefab; //This is a variable to hold the kill listing prefab. [SerializeField] Sprite[] howImages; private void Start() { instance = this; } //To call this function use the following example code //KillFeed.instance.AddNewKillListing("KillerName", "KilledName"); public void AddNewKillListing(string killer, string killed) { GameObject temp = Instantiate(killListingPrefab, transform); temp.transform.SetSiblingIndex(0); KillListing tempListing = temp.GetComponent<KillListing>(); tempListing.SetNames(killer, killed); } //To call this function use the following example code //KillFeed.instance.AddNewKillListingWithHow("KillerName", "KilledName", "Splattered"); public void AddNewKillListingWithHow(string killer, string killed, string how) { GameObject temp = Instantiate(killListingPrefab, transform); temp.transform.SetSiblingIndex(0); KillListing tempListing = temp.GetComponent<KillListing>(); tempListing.SetNamesAndHow(killer, killed, how); } //To call this function use the following example code //KillFeed.instance.AddNewKillListingWithHowImage("KillerName", "KilledName", 0); public void AddNewKillListingWithHowImage(string killer, string killed, int howIndex) { GameObject temp = Instantiate(killListingWithImagePrefab, transform); temp.transform.SetSiblingIndex(0); KillListing tempListing = temp.GetComponent<KillListing>(); tempListing.SetNamesAndHowImage(killer, killed, howImages[howIndex]); } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }