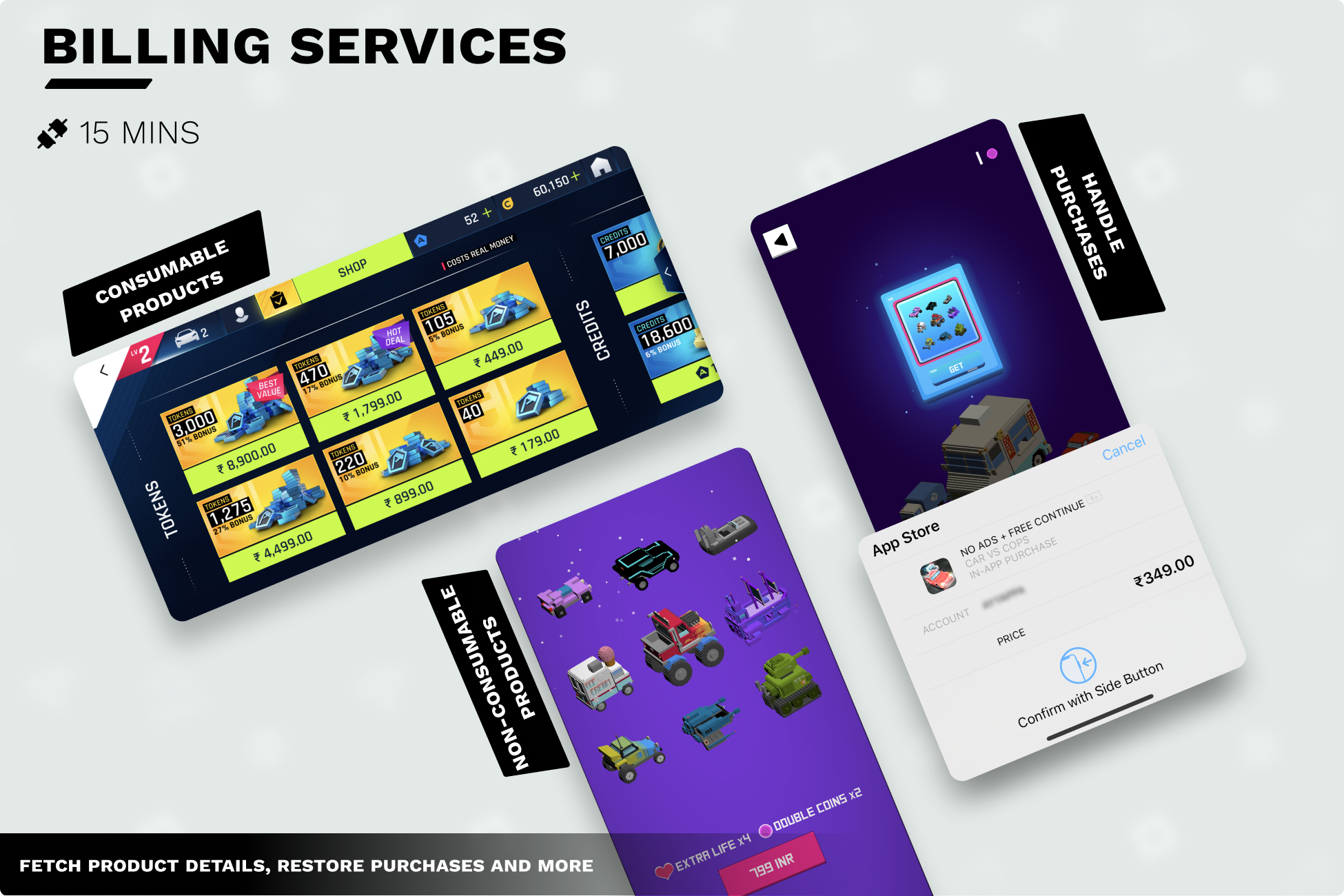
Download
Documentation: https://assetstore.essentialkit.voxelbusters.com/
0:00 Welcome to Billing and IAP
0:21 Overview
1:16 Setup
3:35 iOS
5:03 Android
6:27 Usage and Code
16:30 Setup in Unity
In this tutorial lesson on how to use the Cross Platform Native Plugin 2 in Unity, I will show you how to use the Billing service to create in-app purchases. Adding a store and in-app purchase to your project is one of the best ways to monetize your games. This allows the users to purchase actual content in your game versus monetizing your game with ads only.
IG_Billing.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; using VoxelBusters.EssentialKit; using VoxelBusters.CoreLibrary; public class IG_Billing : MonoBehaviour { [SerializeField] Button OpenStoreButton; //Holds the first button used to enable to Store UI [SerializeField] GameObject storePanel; // Holds the Store UI so we can toggle it on and off [SerializeField] Button[] purchaseButtons; //An Array of all the puchase buttons in our store so we can enable each one if purduct is available // Start is called before the first frame update void Start() { //Check to see if Billing Services in enable in Essental Kit settings if true enable OpenStoreButton and initialize store if (BillingServices.IsAvailable()) { OpenStoreButton.interactable = true; BillingServices.InitializeStore(); } } private void OnEnable() { // register for events BillingServices.OnInitializeStoreComplete += OnInitializeStoreComplete; BillingServices.OnTransactionStateChange += OnTransactionStateChange; BillingServices.OnRestorePurchasesComplete += OnRestorePurchasesComplete; } private void OnDisable() { // unregister from events BillingServices.OnInitializeStoreComplete -= OnInitializeStoreComplete; BillingServices.OnTransactionStateChange -= OnTransactionStateChange; BillingServices.OnRestorePurchasesComplete -= OnRestorePurchasesComplete; } // Register for the BillingServices.OnInitializeStoreComplete event private void OnInitializeStoreComplete(BillingServicesInitializeStoreResult result, Error error) { if (error == null) { // update UI // show console messages var products = result.Products; Debug.Log("Store initialized successfully."); Debug.Log("Total products fetched: " + products.Length); Debug.Log("Below are the available products:"); for (int iter = 0; iter < products.Length; iter++) { //Enable each purchase button in the store for each product that is available and not already purchased purchaseButtons[iter].interactable = !BillingServices.IsProductPurchased(products[iter]); var product = products[iter]; Debug.Log(string.Format("[{0}]: {1}", iter, product)); } } else { Debug.Log("Store initialization failed with error. Error: " + error); } var invalidIds = result.InvalidProductIds; Debug.Log("Total invalid products: " + invalidIds.Length); if (invalidIds.Length > 0) { Debug.Log("Here are the invalid product ids:"); for (int iter = 0; iter < invalidIds.Length; iter++) { Debug.Log(string.Format("[{0}]: {1}", iter, invalidIds[iter])); } } } //This function is set to the on click of each purchase button with the static paramenter set to the products index in Essental Kits settings public void Purchase(int productIndex) { if (BillingServices.CanMakePayments()) { BillingServices.BuyProduct(BillingServices.Products[productIndex]); } } private void OnTransactionStateChange(BillingServicesTransactionStateChangeResult result) { var transactions = result.Transactions; for (int iter = 0; iter < transactions.Length; iter++) { var transaction = transactions[iter]; switch (transaction.TransactionState) { case BillingTransactionState.Purchased: //Check for Receipt. if valid send product to RewardPurchase function if (transaction.ReceiptVerificationState == BillingReceiptVerificationState.Success) { RewardPurchase(transaction.Payment.ProductId); } Debug.Log(string.Format("Buy product with id:{0} finished successfully.", transaction.Payment.ProductId)); break; case BillingTransactionState.Failed: Debug.Log(string.Format("Buy product with id:{0} failed with error. Error: {1}", transaction.Payment.ProductId, transaction.Error)); break; } } } //This function is set to the on click of the Restore Purchase button. public void Restore() { BillingServices.RestorePurchases(); } private void OnRestorePurchasesComplete(BillingServicesRestorePurchasesResult result, Error error) { if (error == null) { var transactions = result.Transactions; Debug.Log("Request to restore purchases finished successfully."); Debug.Log("Total restored products: " + transactions.Length); for (int iter = 0; iter < transactions.Length; iter++) { var transaction = transactions[iter]; //Check for Receipt. If valid send product again to the RewardPurchase function if (transaction.ReceiptVerificationState == BillingReceiptVerificationState.Success) { RewardPurchase(transaction.Payment.ProductId); } Debug.Log(string.Format("[{0}]: {1}", iter, transaction.Payment.ProductId)); } } else { Debug.Log("Request to restore purchases failed with error. Error: " + error); } } //This function is set to the on click of open and close buttons for toggling the Store UI on and off public void ToggleStore() { storePanel.SetActive(!storePanel.activeInHierarchy); } //This function is used to reward to player with everything they have purcahse. void RewardPurchase(string productID) { //Add switch cases for each product you have in your products array switch(productID) { case "remove_ads": Debug.Log("Reward product and save to cloud"); IG_CloudSaving.instance.myCloudData.hasRemoveAds = true; IG_CloudSaving.instance.SaveCloudData(IG_CloudSaving.instance.cloudDataKey, IG_CloudSaving.instance.myCloudData); purchaseButtons[0].interactable = false; break; } } void PlayAd() { if (IG_CloudSaving.instance.myCloudData.hasRemoveAds) return; //Display an ad here! } }