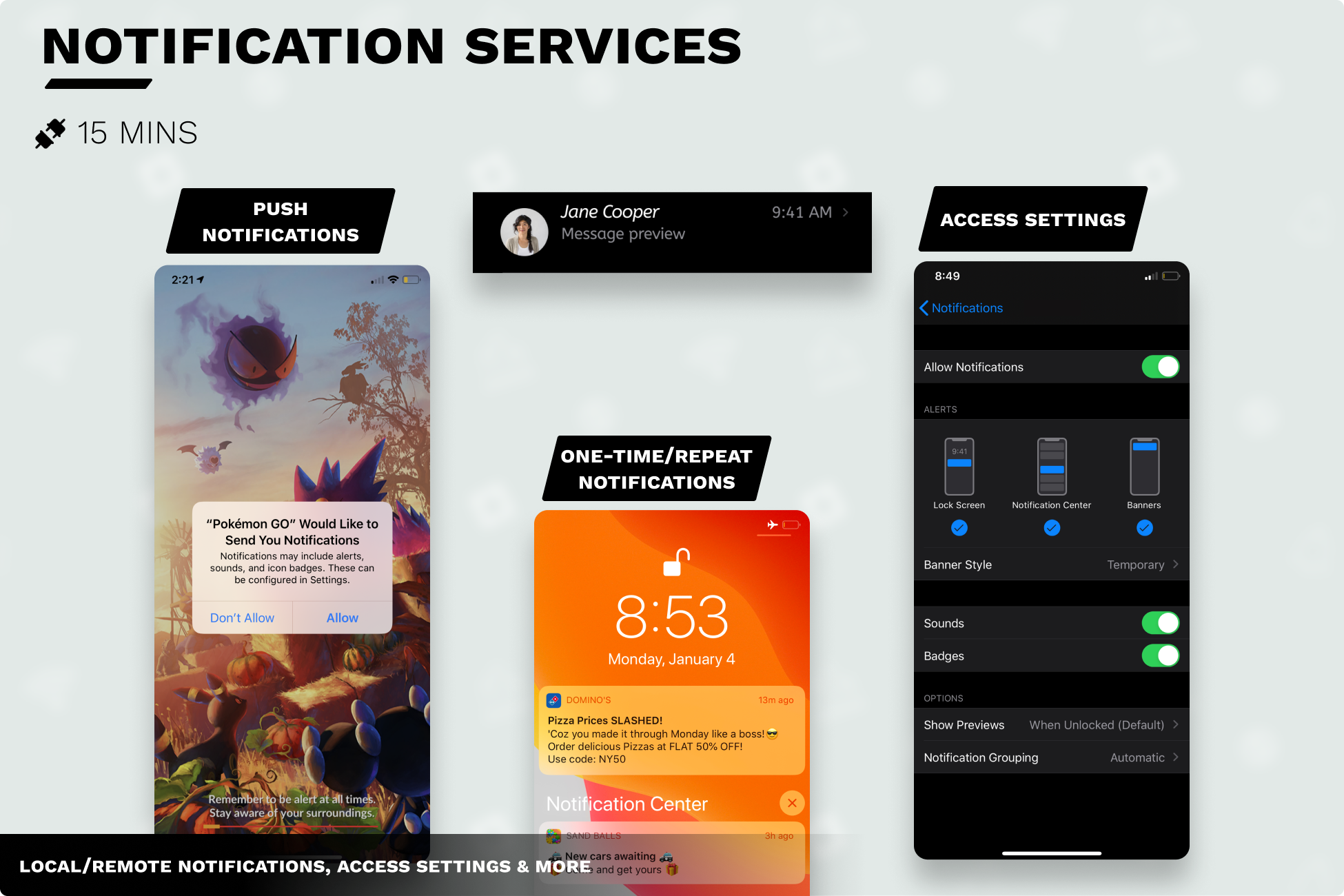
Download
Documentation: https://assetstore.essentialkit.voxelbusters.com/
0:00 Welcome to Notifications CPNP2
0:20 Overview
1:27 Setup
2:45 iOS
3:05 Android
4:29 Usage
For this lesson on how to use the Cross Platform Native Plugins 2 in Unity, I will show you how to use the Notification services. Notifications are important to have in your game as they will help in boosting audience retention. Notification can be used to remind your players to come back and keep playing.
IG_Notifications.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using VoxelBusters.EssentialKit; public class IG_Notifications : MonoBehaviour { public static IG_Notifications instance; NotificationSettings settings; bool isRegistered; // Start is called before the first frame update void Start() { instance = this; VoxelBusters.EssentialKit.NotificationServices.GetSettings((result) => { settings = result.Settings; // update console Debug.Log(settings.ToString()); Debug.Log("Permission status : " + settings.PermissionStatus); }); if(!settings.PushNotificationEnabled) { VoxelBusters.EssentialKit.NotificationServices.UnregisterForPushNotifications(); isRegistered = false; } } //IG_Notifications.instance.CreateLocalNotification("GoldMindFull", "Your gold minds are full!", 3600, false); public void CreateLocalNotification(string notificationID, string notificationTitle, double time, bool canRepeat) { if(settings.PermissionStatus == NotificationPermissionStatus.NotDetermined) { VoxelBusters.EssentialKit.NotificationServices.RequestPermission(NotificationPermissionOptions.Alert | NotificationPermissionOptions.Sound | NotificationPermissionOptions.Badge, callback: (result, error) => { Debug.Log("Request for access finished."); Debug.Log("Notification access status: " + result.PermissionStatus); }); } if(settings.PermissionStatus == NotificationPermissionStatus.Denied) { Utilities.OpenApplicationSettings(); } if (settings.PermissionStatus == NotificationPermissionStatus.Authorized) { INotification notification = NotificationBuilder.CreateNotification(notificationID) .SetTitle(notificationTitle) .SetTimeIntervalNotificationTrigger(time, repeats: canRepeat) .Create(); VoxelBusters.EssentialKit.NotificationServices.ScheduleNotification(notification, (error) => { if (error == null) { Debug.Log("Request to schedule notification finished successfully."); } else { Debug.Log("Request to schedule notification failed with error. Error: " + error); } }); } } private void OnEnable() { VoxelBusters.EssentialKit.NotificationServices.RegisterForPushNotifications((result, error) => { if (error == null) { Debug.Log("Remote notification registration finished successfully. Device token: " + result.DeviceToken); } else { Debug.Log("Remote notification registration failed with error. Error: " + error.Description); } }); isRegistered = VoxelBusters.EssentialKit.NotificationServices.IsRegisteredForPushNotifications(); } private void OnDisable() { if(isRegistered) VoxelBusters.EssentialKit.NotificationServices.UnregisterForPushNotifications(); } }