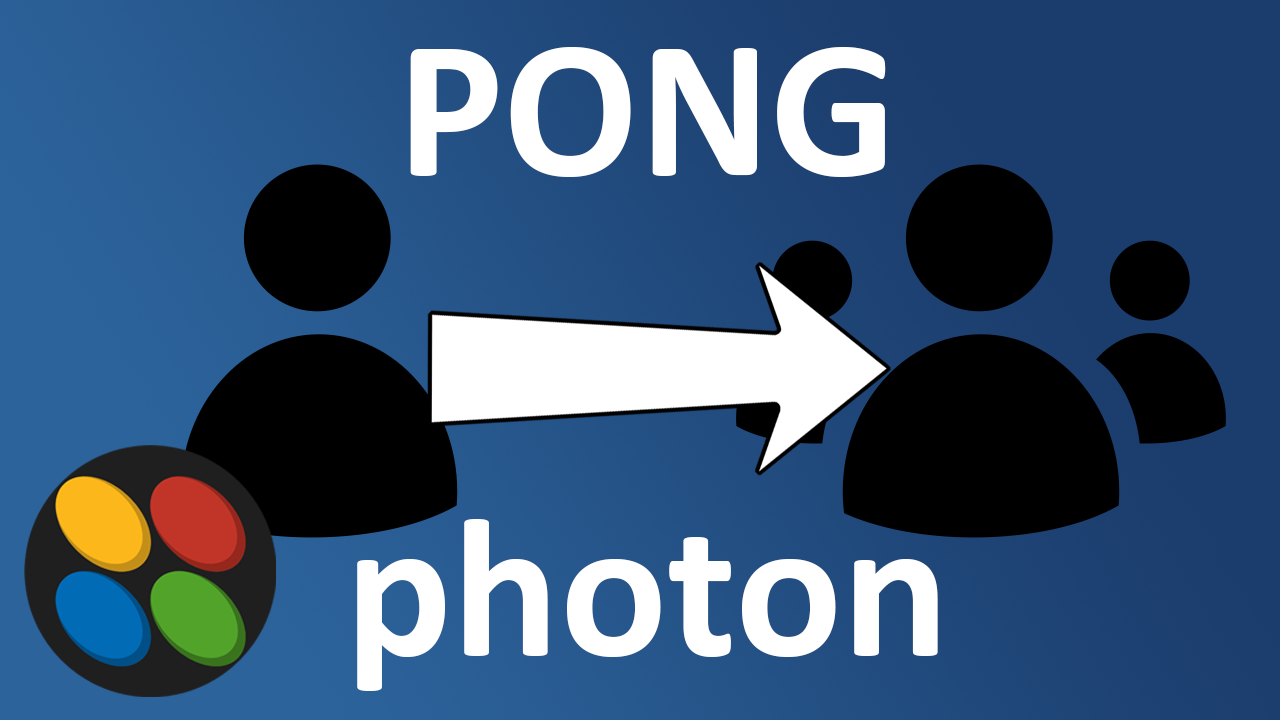
For this lesson on how to make your games multiplayer with Photon PUN in Unity, We will show you how to disconnect for a connected room which will complete our gameplay loop. To do this we want to open up the game controller script inside the script we first need to add the Photon PUN namespace at the top.
Now inside the class, there are two ways that the players can load back to the main menu seen the first is in the update function where we have this if statement where we check for the player’s input when they press the Escape key. Inside this, if statement before we load back to the main menu scene you will want to call the LeaveRoom function.
Now, scroll down where we have this public function called MainMenu, This function is pair the button on our GameOverPanel. Once again before we load to the main menu scene make sure you call the LeaveRoom function.
Now save your script and go back to Unity and test it out.
using UnityEngine; using UnityEngine.UI; using UnityEngine.SceneManagement; using Photon.Pun; public class GameController : MonoBehaviour { public static GameController instance; public bool inPlay = false; bool gameOver = false; public int scoreOne; public int scoreTwo; [SerializeField] int scoreToWin; public Text textOne; public Text textTwo; [SerializeField] GameObject gameOverPanel; [SerializeField] Text winnerText; private void OnEnable() { instance = this; } // Update is called once per frame void Update () { if(inPlay == false && gameOver != true) { if(Input.GetKeyDown(KeyCode.Space)) { inPlay = true; } } if (Input.GetKeyDown(KeyCode.Escape)) { PhotonNetwork.LeaveRoom(); SceneManager.LoadScene(0); } Winner(); } void Winner() { if(!gameOver) { if(scoreOne >= scoreToWin) { gameOver = true; winnerText.text = "Player 1 Wins"; gameOverPanel.SetActive(true); } if(scoreTwo >= scoreToWin) { gameOver = true; winnerText.text = "Player 2 Wins"; gameOverPanel.SetActive(true); } } } public void MainMenu() { PhotonNetwork.LeaveRoom(); SceneManager.LoadScene(0); } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }