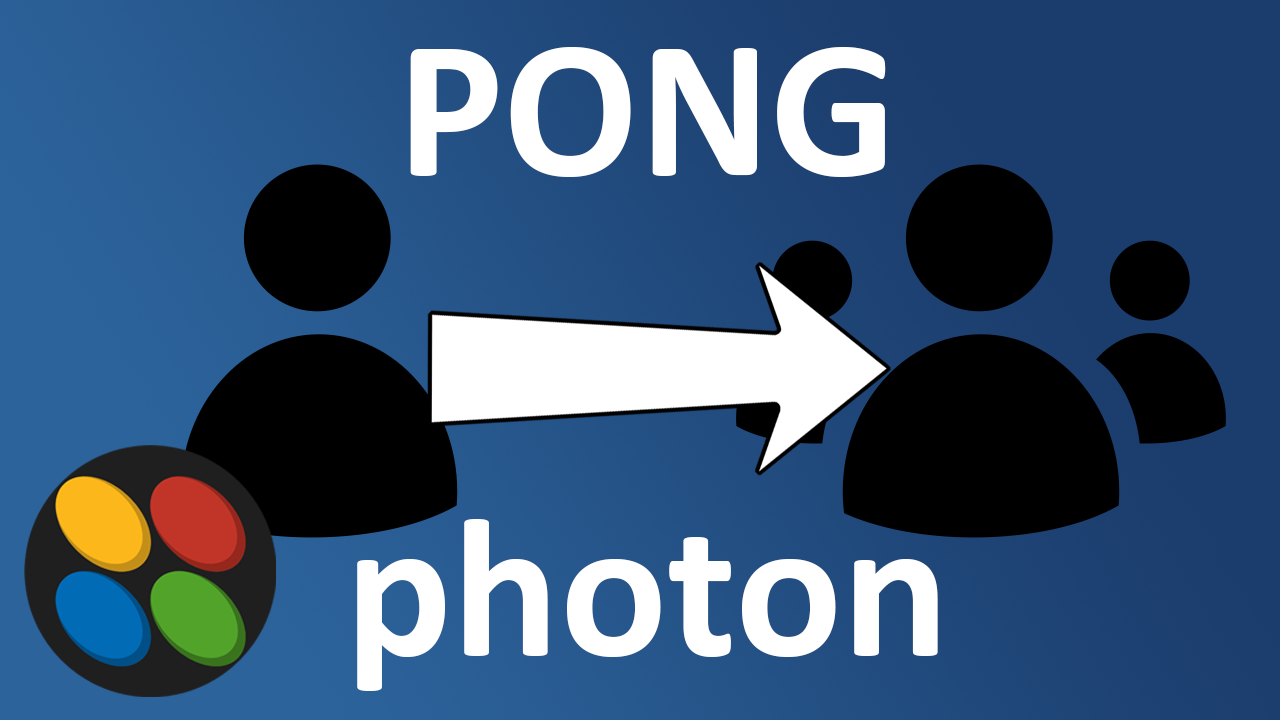
For this lesson of our series on how to make your games multiplayer with Photon’s PUN plugin, we set up the ball prefab so it is networked and synchronized for all connected clients. To get started we want to go to the folder where we have our ball prefab saved and then we want to double click on the ball prefab to open it up. Now like the paddle prefab the first thing that we do is add a photon view component to this object. Next, we will move this ball prefab to inside the Resources folder just in case we decide we want to instantiate more balls into our game.
Now let’s work on synchronizing the movement of our ball and since the ball is moved using physics we want to attach a Photon Rigidbody2d View. Click on add component and then with Photon typed into the search I’m going to click on photon rigidbody2d. We want to have only synchronized velocity selected and will drag this component into the observe component of our Photon View.
Now objects that are owned by the scene are controlled by the master client and so we need to add some conditions to our ball control the script and so open that up. Once this script is opened, add a namespace up at the top which will be using Photon.PUN. Now we’ll add one condition to the beginning of our update function and will check to see if we are not the master client. If we are not then return before doing anything else. This will make it so only the master client moves the ball and that movement will then be synced by the Photon Rigidbody2D View. Save your script and go back to Unity.
Now you can build and test your project.
BallController.cs
using UnityEngine; using System.Collections; using Photon.Pun; public class BallController : MonoBehaviour { Rigidbody2D myRb; bool setSpeed; [SerializeField] float speedUp; float xSpeed; float ySpeed; void Start() { myRb = GetComponent<Rigidbody2D>(); } void Update () { if (!PhotonNetwork.IsMasterClient) return; if(GameController.instance.inPlay == true) { if(!setSpeed) { setSpeed = true; xSpeed = Random.Range(1f, 2f) * (Random.Range(0, 2) * 2 - 1); ySpeed = Random.Range(1f, 2f) * (Random.Range(0, 2) * 2 - 1); } MoveBall(); } } void MoveBall() { myRb.velocity = new Vector2(xSpeed, ySpeed); } void OnCollisionEnter2D(Collision2D other) { if(other.transform.tag =="Wall") { xSpeed = xSpeed*-1; } if (other.transform.tag == "Paddle" ) { ySpeed = ySpeed * -1; if(ySpeed > 0) { ySpeed += speedUp; } else { ySpeed -= speedUp; } if (xSpeed > 0) { xSpeed += speedUp; } else { xSpeed -= speedUp; } } } void OnTriggerEnter2D(Collider2D other) { if(other.tag == "EndOne") { GameController.instance.scoreOne ++; GameController.instance.textOne.text = GameController.instance.scoreOne.ToString(); GameController.instance.inPlay = false; setSpeed = false; myRb.velocity = Vector2.zero; transform.position = Vector2.zero; } else if(other.tag == "EndTwo") { GameController.instance.scoreTwo++; GameController.instance.textTwo.text = GameController.instance.scoreTwo.ToString(); GameController.instance.inPlay = false; setSpeed = false; myRb.velocity = Vector2.zero; transform.position = Vector2.zero; } } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }