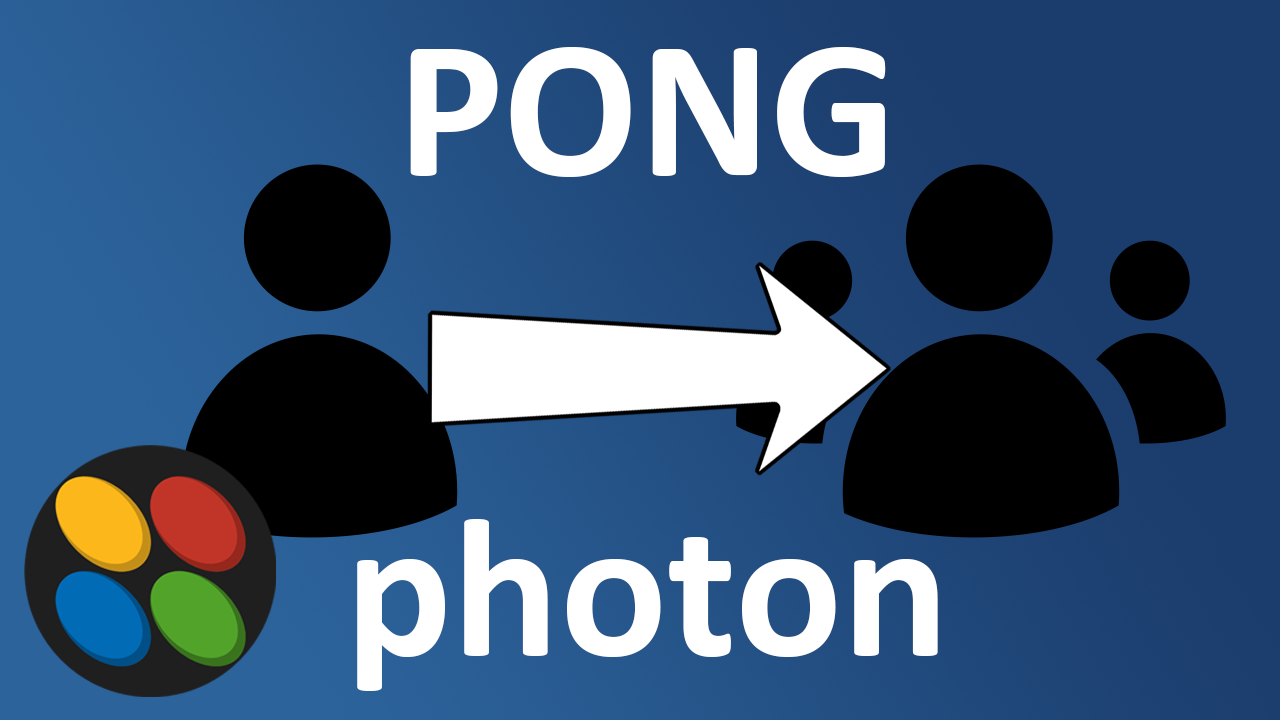
For this lesson on how to make your games multiplayer with Photon PUN in Unity, We will be adding the ability for the players to type in a username. We will then use that username and sync it across the network so that you can see both players’ names. To get started you will first want to add a UI InputField to your QuickStart UI canvas. You can then position and resize this object to fit your liking.
- Canvas
- QuickStartMenu
- Title
- Loading
- QuickStart
- QuickCancel
- – Add the default UI input field object and customize it as you wish.
- QuickStartMenu
Next, we need to create a function that we can pair to this input field so we will open up the MainMenuController script. Inside this script first, add the Photon.PUN namespace. Then create a new public function with a string parameter. Then inside this function set the Photon NickName variable to the string parameter. After which you can then save this script and go back to Unity. Inside Unity, you will need to OnEndEdit event of your object to be this new public function that we just created.
MainMenuController.cs
using UnityEngine; using UnityEngine.SceneManagement; using Photon.Pun; public class MainMenuController: MonoBehaviour { public void Play() { SceneManager.LoadScene(1); } void Update() { if (Input.GetKeyDown(KeyCode.Escape)) { Application.Quit(); } } public void UsernameInput(string username) { PhotonNetwork.NickName = username; } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }
Now that we are reading in and setting the player’s name we can use it in our game. To do this first go to the GameController script. inside this script, we need to add two new Text variables. The first can be called myName and the second can be called theirName. To set this string value of the myName variable, create a Start function. Then inside the start set the string value to the NickName photon variable.
From here we then want to create a public function to set the other player’s name when we receive it. This function could be called SetTheirName and it should have one string parameter. Inside this function just set the string value of the theirName variable to the parameter. Now save this script and go to the PaddleController script.
GameController.cs
using UnityEngine; using UnityEngine.UI; using UnityEngine.SceneManagement; using Photon.Pun; public class GameController : MonoBehaviour { public static GameController instance; public bool inPlay = false; bool gameOver = false; public int scoreOne; public int scoreTwo; [SerializeField] int scoreToWin; public Text textOne; public Text textTwo; [SerializeField] GameObject gameOverPanel; [SerializeField] Text winnerText; [SerializeField] Text myName; [SerializeField] Text theirName; private void OnEnable() { instance = this; } private void Start() { myName.text = PhotonNetwork.NickName; } public void SetTheirName(string nameIn) { theirName.text = nameIn; } // Update is called once per frame void Update () { if(inPlay == false && gameOver != true) { if(Input.GetKeyDown(KeyCode.Space)) { inPlay = true; } } if (Input.GetKeyDown(KeyCode.Escape)) { PhotonNetwork.LeaveRoom(); SceneManager.LoadScene(0); } Winner(); } void Winner() { if(!gameOver) { if(scoreOne >= scoreToWin) { gameOver = true; winnerText.text = "Player 1 Wins"; gameOverPanel.SetActive(true); } if(scoreTwo >= scoreToWin) { gameOver = true; winnerText.text = "Player 2 Wins"; gameOverPanel.SetActive(true); } } } public void MainMenu() { PhotonNetwork.LeaveRoom(); SceneManager.LoadScene(0); } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }
Inside the PaddleController script, we need to create a networked RPC function that will send the local player’s name to the other client. Go ahead and create an RPC function with one parameter of type string. Then inside this function use the singleton of the GameController script to call the SetTheirName function and pass in the parameter. Finally, we need to call this RPC function and we will do that with the Start function right have we rotate the camera. You should call the RPC function within the if statement checking if the local player owns this object. The RPC function should be sent to OthersBufferd and the parameter value should be then local player’s Photon NickName. Now save this script and return to Unity.
PaddleController.cs
using UnityEngine; using Photon.Pun; public class PaddleController : MonoBehaviour { private PhotonView myPV; public string leftKey, rightKey; public float speed; private void Start() { myPV = GetComponent<PhotonView>(); if (myPV.IsMine) { Camera.main.transform.rotation = transform.rotation; myPV.RPC("RPC_SendName", RpcTarget.OthersBuffered, PhotonNetwork.NickName); } } [PunRPC] void RPC_SendName(string nameSent) { GameController.instance.SetTheirName(nameSent); } void Update() { if (myPV.IsMine) { PaddleMovement(); } } void PaddleMovement() { if (Input.GetKey(leftKey) && transform.position.x > -4) { transform.Translate(Vector3.left * Time.deltaTime * speed, Space.Self); } if (Input.GetKey(rightKey) && transform.position.x < 4) { transform.Translate(Vector3.right * Time.deltaTime * speed, Space.Self); } } }
using Photon.Chat; using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class PhotonChatManager : MonoBehaviour, IChatClientListener { #region Setup [SerializeField] GameObject joinChatButton; ChatClient chatClient; bool isConnected; [SerializeField] string username; public void UsernameOnValueChange(string valueIn) { username = valueIn; } public void ChatConnectOnClick() { isConnected = true; chatClient = new ChatClient(this); //chatClient.ChatRegion = "US"; chatClient.Connect(PhotonNetwork.PhotonServerSettings.AppSettings.AppIdChat, PhotonNetwork.AppVersion, new AuthenticationValues(username)); Debug.Log("Connenting"); } #endregion Setup #region General [SerializeField] GameObject chatPanel; string privateReceiver = ""; string currentChat; [SerializeField] InputField chatField; [SerializeField] Text chatDisplay; // Start is called before the first frame update void Start() { } // Update is called once per frame void Update() { if (isConnected) { chatClient.Service(); } if (chatField.text != "" && Input.GetKey(KeyCode.Return)) { SubmitPublicChatOnClick(); SubmitPrivateChatOnClick(); } } #endregion General #region PublicChat public void SubmitPublicChatOnClick() { if (privateReceiver == "") { chatClient.PublishMessage("RegionChannel", currentChat); chatField.text = ""; currentChat = ""; } } public void TypeChatOnValueChange(string valueIn) { currentChat = valueIn; } #endregion PublicChat #region PrivateChat public void ReceiverOnValueChange(string valueIn) { privateReceiver = valueIn; } public void SubmitPrivateChatOnClick() { if (privateReceiver != "") { chatClient.SendPrivateMessage(privateReceiver, currentChat); chatField.text = ""; currentChat = ""; } } #endregion PrivateChat #region Callbacks public void DebugReturn(DebugLevel level, string message) { //throw new System.NotImplementedException(); } public void OnChatStateChange(ChatState state) { if(state == ChatState.Uninitialized) { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } } public void OnConnected() { Debug.Log("Connected"); joinChatButton.SetActive(false); chatClient.Subscribe(new string[] { "RegionChannel" }); } public void OnDisconnected() { isConnected = false; joinChatButton.SetActive(true); chatPanel.SetActive(false); } public void OnGetMessages(string channelName, string[] senders, object[] messages) { string msgs = ""; for (int i = 0; i < senders.Length; i++) { msgs = string.Format("{0}: {1}", senders[i], messages[i]); chatDisplay.text += "\n" + msgs; Debug.Log(msgs); } } public void OnPrivateMessage(string sender, object message, string channelName) { string msgs = ""; msgs = string.Format("(Private) {0}: {1}", sender, message); chatDisplay.text += "\n " + msgs; Debug.Log(msgs); } public void OnStatusUpdate(string user, int status, bool gotMessage, object message) { throw new System.NotImplementedException(); } public void OnSubscribed(string[] channels, bool[] results) { chatPanel.SetActive(true); } public void OnUnsubscribed(string[] channels) { throw new System.NotImplementedException(); } public void OnUserSubscribed(string channel, string user) { throw new System.NotImplementedException(); } public void OnUserUnsubscribed(string channel, string user) { throw new System.NotImplementedException(); } #endregion Callbacks }
Inside Unity, you will need to set the two new variables we create. For these, you can set them to the Player1Keys and Player2Keys objects that we already have from this pong project. We can do this because we no longer need to displayer the controls to the players and they are always the same. You might also want to reposition these objects if necessary.
Now you should have the ability to test your project. You should be able to see the different player’s names on both clients. The local player’s name should be at the bottom of the screen and the other player’s should be at the top